HTML5 Canvas
![]() | What is the the HTML5 canvas, and what can it do? Below you will find some examples and links to summarise its capabilities. http://www.w3schools.com/html/html5_canvas.asp http://diveintohtml5.info/canvas.html http://www.html5canvastutorials.com/ http://code.tutsplus.com/articles/21-ridiculously-impressive-html5-canvas-experiments--net-14210 |
Wikipedia: Canvas Element
The canvas element is part of HTML5 and allows for dynamic, scriptable rendering of 2D shapes and bitmap images. It is a low level, procedural model that updates a bitmap and does not have a built-in scene graph.
Usage
Canvas consists of a drawable region defined in HTML code with height and width attributes. JavaScript code may access the area through a full set of drawing functions similar to those of other common 2D APIs, thus allowing for dynamically generated graphics. Some anticipated uses of canvas include building graphs, animations, games, and image composition.
Examples from www.w3schools.com
Draw a Rectangle
<script> var ctx = document.getElementById("myCanvas1").getContext("2d"); ctx.fillStyle = "#FF0000"; ctx.fillRect(10, 10, 150, 75); </script> |
Draw a Line
<script> var ctx = document.getElementById("myCanvas2").getContext("2d"); ctx.moveTo(10, 10); ctx.lineTo(190, 90); ctx.stroke(); </script> |
Draw a circle
<script> var ctx = document.getElementById("myCanvas3").getContext("2d"); ctx.beginPath(); ctx.arc(95, 50, 40, 0, 2 * Math.PI); ctx.stroke(); </script> |
Draw text
<script> var ctx = document.getElementById("myCanvas4").getContext("2d"); ctx.font = "30px Arial"; ctx.fillText("Hello World", 10, 50); ctx.strokeText("Hello World", 10, 90); </script> |
Draw using gradiant
<script> var ctx = document.getElementById("myCanvas5").getContext("2d"); // Create gradient var grd = ctx.createLinearGradient(0, 0, 200, 0); grd.addColorStop(0, "red"); grd.addColorStop(1, "white"); // Fill with gradient ctx.fillStyle = grd; ctx.fillRect(10, 10, 150, 80); </script> |
Draw an Image
<script> var ctx = document.getElementById("myCanvas6").getContext("2d"); var img = document.getElementById("cupimage"); ctx.drawImage(img, 10, 10); </script> |
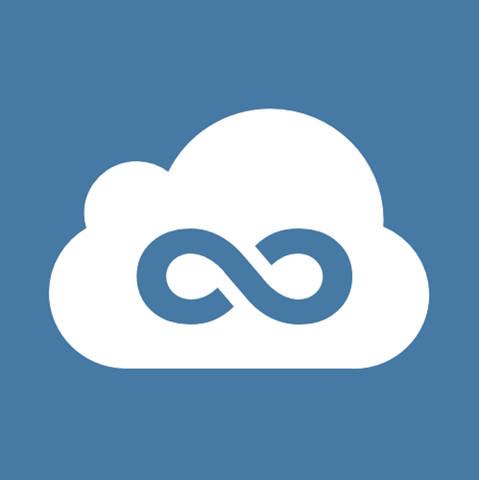