HTML5 Canvas Animation
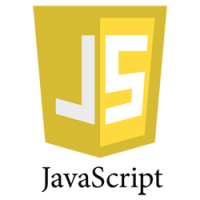
Animating a 2D canvas involves redrawing the canvas via a callback. The basic pattern looks like this:
var requestAnimFrame = (function () {
return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || window.oRequestAnimationFrame || window.msRequestAnimationFrame || function (callback) {
window.setTimeout(callback, 1000 / 60, new Date().getTime());
};
})();function animate() {
draw();
requestAnimationFrame(animate);
}function draw() {
// redraw the next frame here
}window.onload = function (e) {
animate();
}
This demo draws a rectangle and moves it each time it is redrawn:
var canvas = <HTMLCanvasElement>document.getElementById('myCanvas');
var context = canvas.getContext('2d');
var x = 50;
var y = 20;
var vx = 1;function draw() {
x = x + vx;
if (x > 100 || x < 1) {
vx = -vx;
}context.beginPath();
context.rect(x, y, 40, 20);
context.fillStyle = '#8ED6FF';
context.fill();
context.lineWidth = 1;
context.strokeStyle = 'black';
context.stroke();
}